반응형
QT qml의 listview을 이용하여 간단한 리스트 표시하기 - hightlight 포함
listview component는 리스트에 넣을 데이터(model)와 각 리스트 아이템을 어떻게 표시할지에 대한 델리게이트(Delegate)가 꼭 필요합니다. 또한 mouse 클릭한 것에 따라 focus가 변경되는 것을 구현해 봅시다.
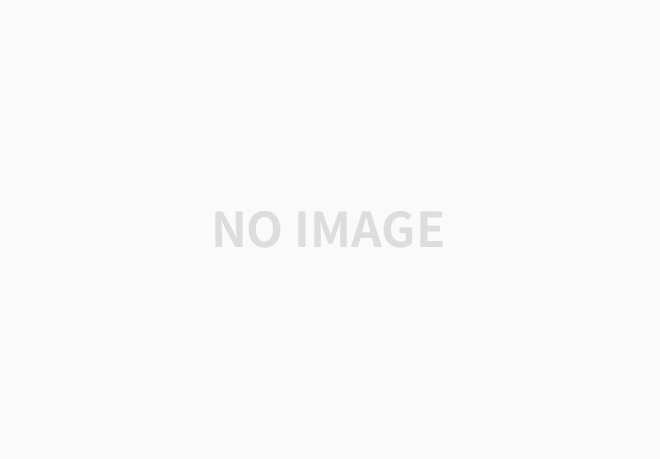
데이터(model) 정의
model은 ListModel component로 생성합니다.
각각의 리스트 아이템은 ListElement로 정의하였고,
과일과 가격을 표시하도록 하기 위해, name과 price 변수를 생성해 값을 지정하였습니다.
ListModel {
id:listmodel
ListElement {
name: "Apple"
price: "1000"
}
ListElement {
name: "Banana"
price: "2000"
}
ListElement {
name: "Grape"
price: "3000"
}
ListElement {
name: "Peach"
price: "4000"
}
ListElement {
name: "Lemon"
price: "5000"
}
}
델리게이트(Delegate) 정의
델리게이트(Delegate)는 리스트의 각각의 아이템을 어떻게 표시할지를 다룹니다.
여기에서는 각 리스트 아이템의 높이는 40으로 넣었고, 과일 이름과 가격을 표시하도록 하였습니다.
또한, Mouse 클릭을 하면 listView의 현재 아이템이 몇번째인지에 대한 currentIndex 변수에 index를 넣도록 하였습니다.
Component {
id:listDelegate
Item {
width: parent.width
height: 40
Column {
Text { text: 'Name:' + name }
Text { text: 'Price:' + price + ' won'}
}
MouseArea {
anchors.fill: parent
onClicked: list.currentIndex = index
}
}
}
하이라이트(Highlight) 정의
리스트의 아이템에 Focus 처리를 하기 위해, 하이라이트를 정의하였습니다.
배경은 회색으로 표시하도록 하였고, 마우스로 클릭한 아이템의 과일 이름 문구를 표시하도록 하였습니다.
문구의 색상은 흰색으로 표시하도록 하였습니다.
Component{
id : listhightlight
Rectangle {
color: 'grey'
Text {
anchors.centerIn: parent
text: 'Hello ' + listmodel.get(list.currentIndex).name
color: 'white'
}
}
}
전체코드
import QtQuick 2.12
import QtQuick.Window 2.12
import QtQuick.Controls 2.12
Window {
width: 640
height: 480
visible: true
ListModel {
id:listmodel
ListElement {
name: "Apple"
price: "1000"
}
ListElement {
name: "Banana"
price: "2000"
}
ListElement {
name: "Grape"
price: "3000"
}
ListElement {
name: "Peach"
price: "4000"
}
ListElement {
name: "Lemon"
price: "5000"
}
}
Component {
id:listDelegate
Item {
width: parent.width
height: 40
Column {
Text { text: 'Name:' + name }
Text { text: 'Price:' + price + ' won'}
}
MouseArea {
anchors.fill: parent
onClicked: list.currentIndex = index
}
}
}
Component{
id : listhightlight
Rectangle {
color: 'grey'
Text {
anchors.centerIn: parent
text: 'Hello ' + listmodel.get(list.currentIndex).name
color: 'white'
}
}
}
ListView {
id: list
anchors.fill: parent
model: listmodel
delegate:listDelegate
highlight:listhightlight
focus: true
onCurrentItemChanged: console.log(model.get(list.currentIndex).name + ' selected')
}
}
반응형
'프로그래밍 > QT_QML' 카테고리의 다른 글
QT qml의 slider를 이용해 Rectangle 색상 변경하기 (0) | 2020.07.30 |
---|---|
QT qml의 popup 표시하고 timer를 이용해 팝업 닫기 (0) | 2020.07.29 |
QT qml의 TextField, Button을 이용한 Label 문구 업데이트 하기 (0) | 2020.07.25 |
QT - Widget, Console, Quick 프로젝트 생성하여 Hello world 출력하기 (0) | 2020.07.25 |
QT Creator 설치하기 - 윈도우 용 (0) | 2020.07.23 |